golang中的枚举类型
- iota是golang的常量计数器,只能在常量表达式中使用。
- iota在const关键字出现时被重置为0(const内部的第一行之前),const中每新增一行常量声明将使iota计数一次(iota可理解为const语句块中的行索引)。
- 使用iota能简化定义,在定义枚举时很有用。
iota的使用场景示例:
- iota只能在常量的表达式中使用
fmt.Println(iota)
//编译错误: undefined: iota
- 每次 const 出现时,都会让 iota 初始化为0
const a = iota // a=0
const (
b = iota //b=0
c //c=1 相当于c=iota
)
- 自增长常量经常包含一个自定义枚举类型,允许依靠编译器完成自增设置
type Stereotype int
const (
TypicalNoob Stereotype = iota // 0
TypicalHipster // 1 TypicalHipster = iota
TypicalUnixWizard // 2 TypicalUnixWizard = iota
TypicalStartupFounder // 3 TypicalStartupFounder = iota
)
- 可跳过的值
//如果两个const的赋值语句的表达式是一样的,那么可以省略后一个赋值表达式。
type AudioOutput int
const (
OutMute AudioOutput = iota // 0
OutMono // 1
OutStereo // 2
_
_
OutSurround // 5
)
- 位掩码表达式
type Allergen int
const (
IgEggs Allergen = 1 << iota // 1 << 0 which is 00000001
IgChocolate // 1 << 1 which is 00000010
IgNuts // 1 << 2 which is 00000100
IgStrawberries // 1 << 3 which is 00001000
IgShellfish // 1 << 4 which is 00010000
)
- 定义数量级
type ByteSize float64
const (
_ = iota // ignore first value by assigning to blank identifier
KB ByteSize = 1 << (10 * iota) // 1 << (10*1)
MB // 1 << (10*2)
GB // 1 << (10*3)
TB // 1 << (10*4)
PB // 1 << (10*5)
EB // 1 << (10*6)
ZB // 1 << (10*7)
YB // 1 << (10*8)
)
- 定义在一行的情况
const (
Apple, Banana = iota + 1, iota + 2
Cherimoya, Durian // = iota + 1, iota + 2
Elderberry, Fig //= iota + 1, iota + 2
)
iota 在下一行增长,而不是立即取得它的引用
// Apple: 1
// Banana: 2
// Cherimoya: 2
// Durian: 3
// Elderberry: 3
// Fig: 4
- 中间插队
const (
i = iota
j = 3.14
k = iota
l
)
//那么打印出来的结果是 i=0,j=3.14,k=2,l=3
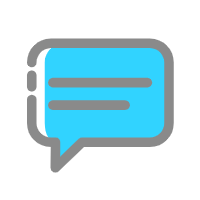
0 评论